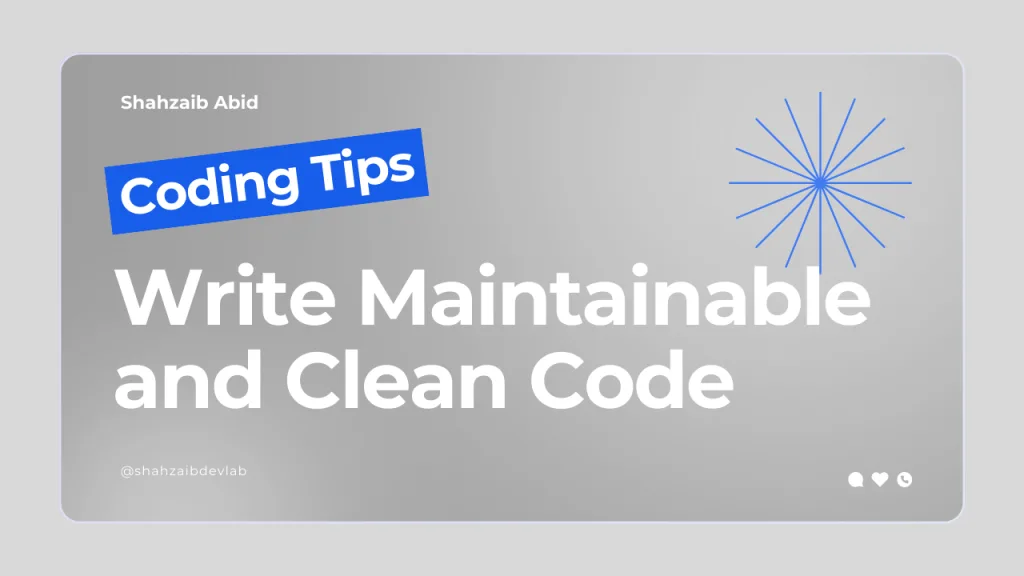
🚀 Writing clean and maintainable code is not just a skill—it’s a superpower for developers. If you want to build scalable and bug-free applications, you need to master the art of clean coding. In this article, we’ll break down the essential principles that will elevate your coding game! 💡
Why Does Clean Code Matter?
- Faster Debugging: Easier to understand means easier to fix.
- Better Collaboration: Other developers can easily work on your code.
- Future-Proofing: Scalable and adaptable for future changes.
Core Principles of Writing Clean and Maintainable Code
1. Keep It Simple (KISS)
Avoid overcomplicating your code. Simplicity enhances readability and makes debugging effortless.
Example:
// ❌ Overcomplicated
double calculateArea(double width, double height) {
return width * height;
}
// ✅ Simple and Clear
double calculateArea(double width, double height) => width * height;
2. Don’t Repeat Yourself (DRY)
Eliminate redundant code by using functions, classes, or reusable components.
Example:
// ❌ Repetitive Code
void printWelcome() {
print("Welcome to our app!");
}
// ✅ DRY Principle
void printMessage(String message) {
print(message);
}

3. Use Meaningful Names
Variable and function names should describe their purpose clearly.
Example:
// ❌ Bad Naming
int a = 10;
// ✅ Good Naming
int itemCount = 10;
4. Single Responsibility Principle (SRP)
Each function or class should have only one responsibility to keep your code modular.
Example:
// ❌ Violates SRP
class User {
void saveUser() {
// Save user logic
}
void sendEmail() {
// Send email logic
}
}
// ✅ Follows SRP
class UserRepository {
void saveUser() {
// Save user logic
}
}
class EmailService {
void sendEmail() {
// Send email logic
}
}
5. Write Reusable and Modular Code
Break your code into smaller, reusable components.
Example:
// ✅ Modular Code
class Button extends StatelessWidget {
final String text;
final VoidCallback onPressed;
Button({required this.text, required this.onPressed});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: onPressed,
child: Text(text),
);
}
}
6. Write Tests
Testing ensures reliability and prevents regressions.
Example:
void main() {
test('Sum function should return correct sum', () {
expect(sum(2, 3), equals(5));
});
}
7. Write Self-Documenting Code
Good code should be easy to understand without requiring excessive comments. Self-documenting code improves readability and reduces the need for additional explanations.
Example:
// ❌ Hard to understand
dbl c(double p, double r, double t) {
return p * r * t;
}
// ✅ Self-documenting code
double calculateInterest(double principal, double rate, double time) {
return principal * rate * time;
}
Using descriptive variable and function names ensures that your code speaks for itself. This makes collaboration and future maintenance easier.
8. Keep Functions Short and Focused
Functions should be small and do only one thing well. If a function grows too large, consider breaking it into smaller helper functions.
Example:
// ❌ Too long function
void processUser() {
validateUser();
saveUserToDatabase();
sendWelcomeEmail();
logActivity();
}
// ✅ Short and focused functions
void processUser() {
validateUser();
saveUser();
notifyUser();
}

Final Thoughts
By following these principles, you’ll write clean, maintainable, and scalable code that makes development smoother. Ready to dive deeper? 🚀
💬 Have an idea? Want to launch your own product? Let’s talk! Drop me a message, and let’s build something great together! 💡🔥
Follow me on LinkedIn for the latest updates.