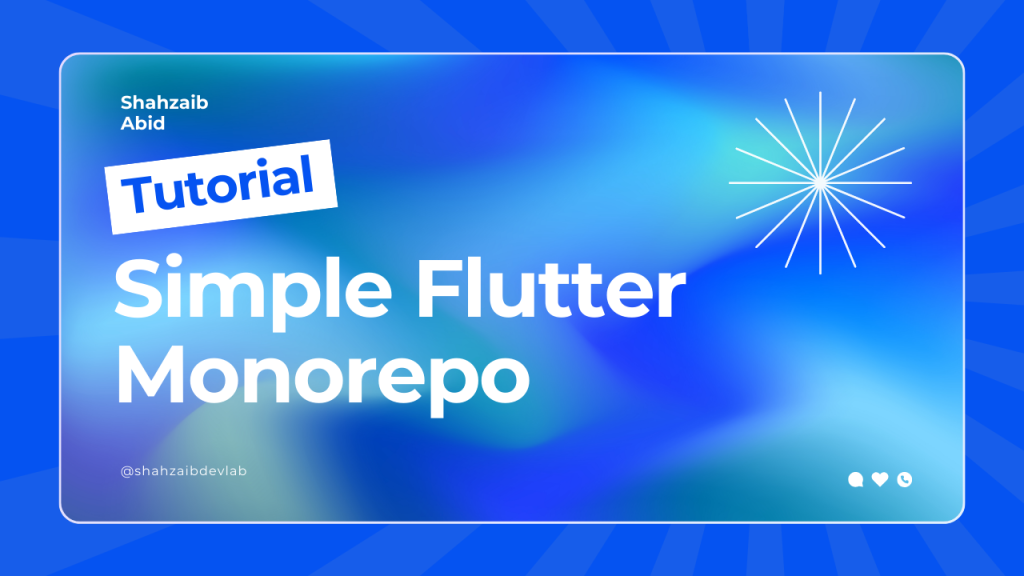
Are you looking to set up a Flutter monorepo but want to streamline the process without unnecessary complexity? In this tutorial, I’ll guide you step by step on how to create a simple Flutter monorepo using Melos. Melos will handle the heavy lifting, making dependency management and automation a breeze, and helping you maintain multiple apps and shared packages in one clean structure.
What is a Flutter Monorepo?
A Flutter monorepo is a repository structure that contains multiple Flutter applications and shared packages. This setup makes it easier to share code between apps, manage dependencies centrally, and keep your projects organized.
Why Use Melos in a Flutter Monorepo?
Melos is a tool designed for managing monorepos efficiently. It helps automate tasks like dependency resolution, executing commands across multiple packages/apps, and handling versioning. By using Melos in your Flutter monorepo, you can:
- Streamline dependency management.
- Avoid redundancy across multiple projects.
- Automate repetitive tasks.
This tutorial will show you how to set up and use Melos for your Flutter monorepo.
Step 1: Setting Up the Flutter Monorepo Structure
Before diving into Melos, let’s set up a basic monorepo structure for your Flutter projects.
1. Create your monorepo folder:
mkdir flutter-monorepo
cd flutter-monorepo
2. Create the directory structure:
flutter-monorepo/
├── apps/
│ ├── app_one/ # First Flutter app
│ ├── app_two/ # Second Flutter app
├── packages/
│ ├── my_shared_package/ # Shared Dart/Flutter package
Step 2: Initialize a Flutter Package
We need to create a shared package that both of your Flutter apps will use.
1. Create the shared package my_shared_package
:
Run the following command to create the package:
flutter create --template=package packages/my_shared_package
2. Modify the shared package:
Open the packages/my_shared_package/lib/my_shared_package.dart
file and add a simple function:
library my_shared_package;
String sharedFunction() => "Hello from the shared package!";
Step 3: Create Two Flutter Apps
Now, let’s create two Flutter apps that will utilize the shared package.
1. Create the first app (app_one
):
Run the following command to create your first Flutter app:
flutter create apps/app_one
2. Modify the app to use the shared package:
Open apps/app_one/pubspec.yaml
and add the shared package as a dependency:
dependencies:
flutter:
sdk: flutter
my_shared_package:
path: ../../packages/my_shared_package
3. Use the shared function in the app:
In apps/app_one/lib/main.dart
, modify the code to display a message from the shared package:
import 'package:flutter/material.dart';
import 'package:my_shared_package/my_shared_package.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text("App One")),
body: Center(child: Text(sharedFunction())),
),
);
}
}
4. Create the second app (app_two
):
Similarly, create the second Flutter app:
flutter create apps/app_two
5. Add the shared package to app_two
:
Modify the pubspec.yaml
file in app_two
just like we did for app_one
:
dependencies:
flutter:
sdk: flutter
my_shared_package:
path: ../../packages/my_shared_package
6. Use the shared function in the second app:
In apps/app_two/lib/main.dart
, display the message from the shared package:
import 'package:flutter/material.dart';
import 'package:my_shared_package/my_shared_package.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text("App Two")),
body: Center(child: Text(sharedFunction())),
),
);
}
}
Step 4: Add Melos to Your Monorepo
Now that your monorepo structure is in place, let’s integrate Melos to manage the dependencies and tasks across multiple apps and packages.
1. Create a pubspec.yaml
file at the root of your monorepo (if it doesn’t already exist):
name: flutter_monorepo
description: Monorepo for managing Flutter apps and packages.
version: 1.0.0
environment:
sdk: ">=3.0.0 <4.0.0"
dependencies:
# Add any general dependencies needed for the monorepo.
dev_dependencies:
melos: ^3.0.0 # Add Melos as a development dependency
2. Install Melos locally:
Run the following command to install Melos in your monorepo:
flutter pub get
Step 5: Create melos.yaml
for Task Automation
In your root directory, create a melos.yaml
file to configure Melos for managing the monorepo.
Here’s an example melos.yaml
file:
name: flutter_monorepo
packages:
- "apps/*"
- "packages/*"
scripts:
bootstrap: |
# Runs flutter pub get in all apps and packages
melos exec -- flutter pub get
run_all_apps: |
# Runs both apps sequentially
(cd apps/app_one && flutter run) &
(cd apps/app_two && flutter run)
1. bootstrap
: This script installs dependencies for all packages and apps using flutter pub get
.
2. run_all_apps
: This script runs both apps in parallel.
Step 6: Run Melos Commands
1. Install Melos Globally:
Run the following command to install melos globally:
dart pub global activate melos
2. Bootstrap the Monorepo:
Run the following command to install dependencies for all apps and packages:
melos bootstrap
3. Run Both Apps:
After bootstrapping, run both apps using the run_all_apps
script:
melos run run_all_apps
Both apps should now launch simultaneously, and the shared package should be linked correctly.
Conclusion
In this tutorial, we set up a simple Flutter monorepo with two apps and one shared package. We integrated Melos to automate tasks like dependency resolution and app running. By following these steps, you now have a scalable structure that makes managing multiple apps and shared code much simpler.
As your project grows, you can leverage Melos for more advanced workflows, like parallel execution of tasks, versioning, and more. This simple approach provides you with all the foundational tools to manage a Flutter monorepo effectively.
Let’s Connect!
Stay ahead of app development trends with expert insights and practical tips:
Follow me on LinkedIn for the latest updates.
Need a professional mobile app or website for your business? Let’s build something great together at KEYBOTIX SOLUTIONS.
Get the Full Code Example on GitHub
Want to see the Flutter Monorepo in action? Dive into the complete project and explore the setup firsthand. Clone, experiment, and integrate it into your own workflow!